Generate Key When I Map Array Javascript
Feb 10, 2020 Arrays in JavaScript are very commonly used DataStructure. It can be very handy to know your way around Arrays. Their non-mutating nature allows us to create new Arrays in different ways. Map function will loop over items and return a new array of mapped Items. You can create array of Keys or Values of any Object with. Create Key Value Pair Array Using Javascript, Our today's article is simple but demanding. Most of the new or experience java-script developer required below code. In this code snippet I have define java-script array values are in the form of key and value. Jul 20, 2013 Sequences using JavaScript Array. Jul 20, 2013 5 min read #es6 #javascript #tip. Generating a sequence is a common programming task. This is rather easy to achieve using a straightforward loop. With JavaScript however, there exists a more functional variant using the powerful Array object.
- Map To Array Javascript
- Generate Key When I Map Array Javascript In Word
- Javascript Generate Array Of Numbers
- Generate Key When I Map Array Javascript Code
- Generate Key When I Map Array Javascript In Java
- Javascript Key Value Array
- Generate Key When I Map Array Javascript Download
This article was peer reviewed by Chris Perry and Marcello La Rocca. Thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
The length
property of Array
objects is one that many who are relatively new to JavaScript do not understand. Many mistakenly believe that the length tells you exactly how many entries there are in an array whereas this is only true of some arrays. Some beginners do not even realize that length
is a writable property of arrays. To clarify just exactly how the length
property works, let’s take a look at what happens when we either change its value ourselves or run something that updates the array that also results in the length changing.
Let’s start at the beginning. A JavaScript array has a property called length
and optionally has numbered properties with names between 0 and 4294967294 inclusive. It also has a number of methods for manipulating the properties some of which we will look at as a part of our examination of how the length property works. Note that JavaScript does not support associative arrays and so while you can add named properties to an array, they do not form a part of the array and will be ignored by all the array methods. They also will not affect the length.
To make it easier to show exactly what happens to the array properties as we process various statements, we will run the following function after each piece of code. This will log the length of the array and all of the numbered properties to the browser’s console.
Creating an Array
We will begin by looking at different ways to create an array in JavaScript. The first two of these examples create arrays where only the length is set and there are no numbered entries at all. The second two create numbered entries from 0 to one less than the length.
An array where the length is greater than the amount of numbered properties is known as a sparse array while one with the length equal to the number of numbered properties is a dense array.
Note that the array literal notation (where you define a new array using just empty brackets) is preferred when creating new arrays.
The array methods that process the numbered properties (forEach
in our case) will only process those that exist. If you instead process the array using a for
or while
loop then the loop will also attempt to process those properties that don’t exist and the array will identify those entries that don’t exist as being undefined
. Your code would then be unable to distinguish between the last of the above examples and the first two. You should always use the array methods for processing an array where you are not certain that you are dealing with a dense array.
Changing the Length
The following examples look at what happens if we set a new length for the array that is less than the current length.
Note that when creating an array using []
notation each entry consists of a value followed by a comma. Where the value is omitted then no property is created for that position. The last comma may only be omitted if there is a value supplied for that property as otherwise the length will be reduced by one.
Removing Entries
JavaScript provides three methods pop, shift and splice that can remove entries from the array and which therefore reduce the length of the array. In each case the value (or values) removed are returned by the call.
Adding Entries
We can add a new entry into an array simply by specifying a position in the array for which a numbered property does not yet exist. We can also use one of the three methods JavaScript provides (push, unshift and splice) for inserting new entries and, where necessary, moving the old ones.
Replacing Entries
Where we assign a new value to an entry that already exists, then that entry simply gets a new value and the rest of the array is unaffected. Also by combining the variants of the splice()
method that we have already looked at we can replace existing entries or fill gaps in the array.
Conclusion
The above examples should have given you a better idea of how the length
property of an array works. This can be greater or equal to the number of entries in the array. Where it is equal we have a dense array and where it is greater we have a sparse array. Exactly what a particular array method does can depend on whether there is actually a property corresponding to a given position in a sparse array. If we change the length of an array it removes any numbered properties in the array that are in positions that are greater than the new length. If the length was equal to the amount of numbered properties and we increase the length then we convert a dense array to a sparse one. The array methods for deleting and adding properties in the array will move the existing entries around where necessary and will also retain and move any gaps between the properties.
JavaScript arrays are used to store multiple values in a single variable.
Example
Try it Yourself »What is an Array?
An array is a special variable, which can hold more than one value at a time.
If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
var car2 = 'Volvo';
var car3 = 'BMW';
However, what if you want to loop through the cars and find a specific one? And what if you had not 3 cars, but 300?
The solution is an array!
An array can hold many values under a single name, and you can access the values by referring to an index number.
Creating an Array
Using an array literal is the easiest way to create a JavaScript Array.
Syntax:
Example
Try it Yourself »Spaces and line breaks are not important. A declaration can span multiple lines:
Example
Try it Yourself »Using the JavaScript Keyword new
The following example also creates an Array, and assigns values to it:
Example
Try it Yourself »The two examples above do exactly the same. There is no need to use new Array()
.
For simplicity, readability and execution speed, use the first one (the array literal method).
Access the Elements of an Array
You access an array element by referring to the index number.
This statement accesses the value of the first element in cars
:
Example
document.getElementById('demo').innerHTML = cars[0];
Note: Array indexes start with 0.
[0] is the first element. [1] is the second element.
Changing an Array Element
This statement changes the value of the first element in cars
:
Example
cars[0] = 'Opel';
document.getElementById('demo').innerHTML = cars[0];
Access the Full Array
With JavaScript, the full array can be accessed by referring to the array name:
Example
document.getElementById('demo').innerHTML = cars;
Arrays are Objects
Arrays are a special type of objects. The typeof
operator in JavaScript returns 'object' for arrays.
But, JavaScript arrays are best described as arrays.
Arrays use numbers to access its 'elements'. In this example, person[0]
returns John:
Array:
Try it Yourself »Objects use names to access its 'members'. In this example, person.firstName
returns John:
Object:
Array Elements Can Be Objects
JavaScript variables can be objects. Arrays are special kinds of objects.
Because of this, you can have variables of different types in the same Array.
You can have objects in an Array. You can have functions in an Array. You can have arrays in an Array:
What’s the catch?Our partners regularly give us a large number of free CD keys which allows our team to offer you totally free keys every moment via keygen tool.
myArray[1] = myFunction;
myArray[2] = myCars;
Array Properties and Methods
The real strength of JavaScript arrays are the built-in array properties and methods:
Examples
var y = cars.sort(); // The sort() method sorts arrays
Array methods are covered in the next chapters.
The length Property
The length
property of an array returns the length of an array (the number of array elements).
Example
fruits.length; // the length of fruits is 4
The length
property is always one more than the highest array index.
Accessing the First Array Element
Example
var first = fruits[0];
Accessing the Last Array Element
Example
var last = fruits[fruits.length - 1];
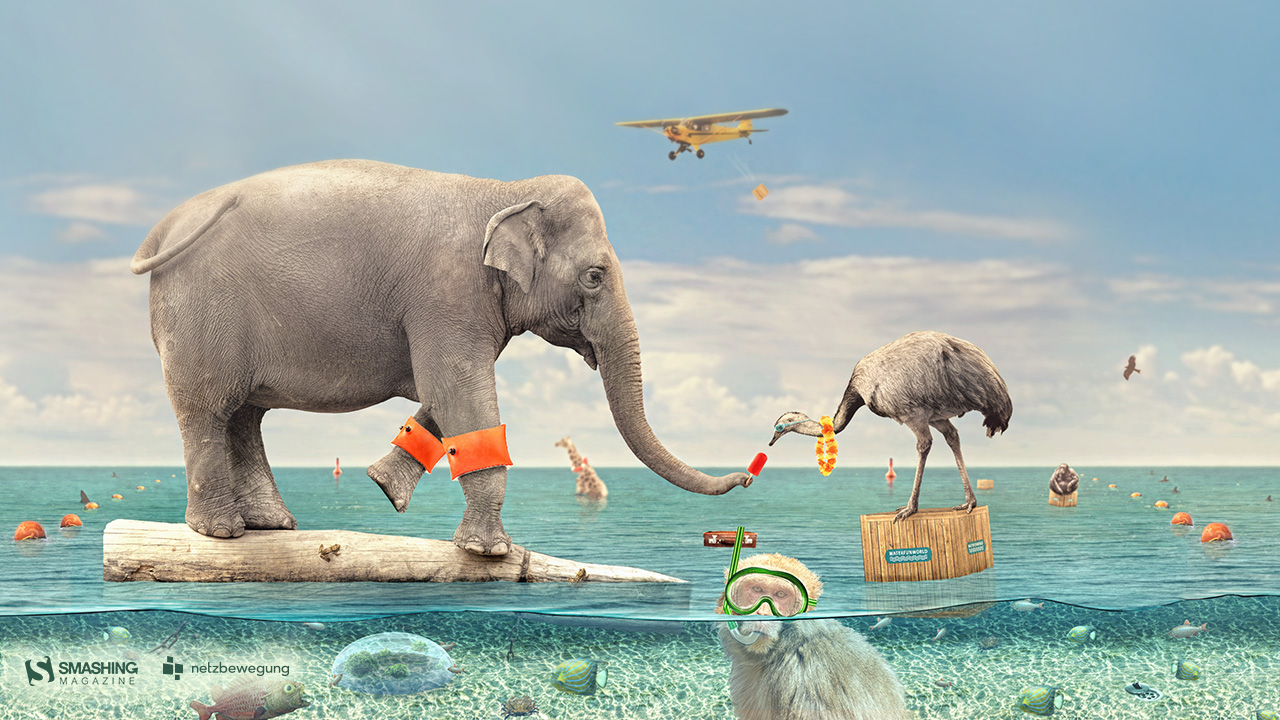
Looping Array Elements
The safest way to loop through an array, is using a for
loop:
Example
fruits = ['Banana', 'Orange', 'Apple', 'Mango'];
fLen = fruits.length;
text = '<ul>';
for (i = 0; i < fLen; i++) {
text += '<li>' + fruits[i] + '</li>';
}
text += '</ul>';
You can also use the Array.forEach()
function:
Example
fruits = ['Banana', 'Orange', 'Apple', 'Mango'];
text = '<ul>';
fruits.forEach(myFunction);
text += '</ul>';
function myFunction(value) {
text += '<li>' + value + '</li>';
}
Adding Array Elements
The easiest way to add a new element to an array is using the push()
method:
Dark souls prepare to die edition cd key generator. Out of these cookies, the cookies that are categorized as necessary are stored on your browser as they are essential for the working of basic functionalities of the website. These cookies will be stored in your browser only with your consent. This website uses cookies to improve your experience while you navigate through the website. We also use third-party cookies that help us analyze and understand how you use this website.
Example
fruits.push('Lemon'); // adds a new element (Lemon) to fruits
New element can also be added to an array using the length
property:
Example
fruits[fruits.length] = 'Lemon'; // adds a new element (Lemon) to fruits
WARNING !
Adding elements with high indexes can create undefined 'holes' in an array:
Example
fruits[6] = 'Lemon'; // adds a new element (Lemon) to fruits
Associative Arrays
Many programming languages support arrays with named indexes.
Arrays with named indexes are called associative arrays (or hashes).
JavaScript does not support arrays with named indexes.
In JavaScript, arrays always use numbered indexes.
Example
person[0] = 'John';
person[1] = 'Doe';
person[2] = 46;
var x = person.length; // person.length will return 3
var y = person[0]; // person[0] will return 'John'
WARNING !!
If you use named indexes, JavaScript will redefine the array to a standard object.
After that, some array methods and properties will produce incorrect results.
Example:
person['firstName'] = 'John';
person['lastName'] = 'Doe';
person['age'] = 46;
var x = person.length; // person.length will return 0
var y = person[0]; // person[0] will return undefined
The Difference Between Arrays and Objects
In JavaScript, arrays use numbered indexes.
In JavaScript, objects use named indexes.
Arrays are a special kind of objects, with numbered indexes.
When to Use Arrays. When to use Objects.
- JavaScript does not support associative arrays.
- You should use objects when you want the element names to be strings (text).
- You should use arrays when you want the element names to be numbers.
Avoid new Array()
There is no need to use the JavaScript's built-in array constructor new
Array().
Use []
instead.
These two different statements both create a new empty array named points:
var points = []; // Good
These two different statements both create a new array containing 6 numbers:
Map To Array Javascript
var points = [40, 100, 1, 5, 25, 10]; // Good
The new
keyword only complicates the code. It can also produce some unexpected results:
What if I remove one of the elements?
How to Recognize an Array
A common question is: How do I know if a variable is an array?
The problem is that the JavaScript operator typeof
returns 'object
':
typeof fruits; // returns object
The typeof operator returns object because a JavaScript array is an object.
Solution 1:
To solve this problem ECMAScript 5 defines a new method Array.isArray()
:
Generate Key When I Map Array Javascript In Word
The problem with this solution is that ECMAScript 5 is not supported in older browsers.
Solution 2:
To solve this problem you can create your own isArray()
function:
return x.constructor.toString().indexOf('Array') > -1;
}
Javascript Generate Array Of Numbers
Try it Yourself »Generate Key When I Map Array Javascript Code
The function above always returns true if the argument is an array.
Or more precisely: it returns true if the object prototype contains the word 'Array'.
Solution 3:
Generate Key When I Map Array Javascript In Java
The instanceof
operator returns true if an object is created by a given constructor:
Javascript Key Value Array
fruits instanceof Array; // returns true